Unraveling the Mysteries of Python’s Impressive MetaClasses
Exploring the power and potential pitfalls of Python’s MetaClasses
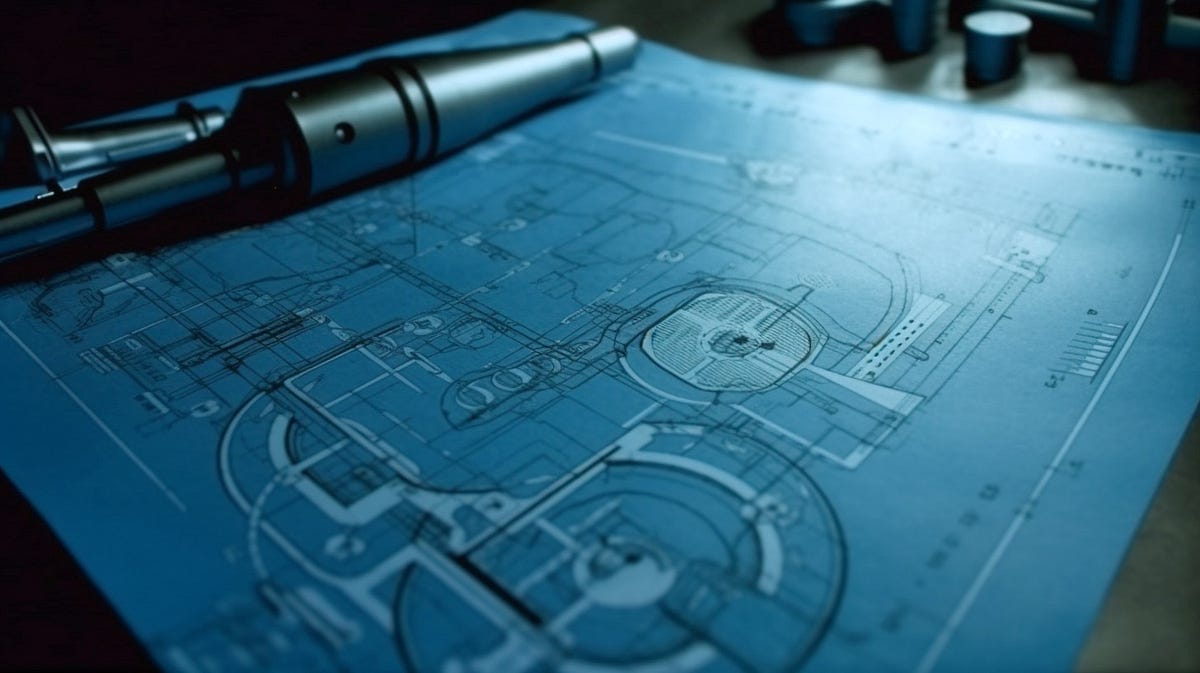
Alex squinted at his laptop screen, his fifth cup of coffee gone cold. He was working on an intricate component of Streamfinity’s Python project.
The piece Alex was laboring over was a crucial part of the project. It was a system that needed to create several objects, each representing a unique digital asset in its platform.
Jordan, a fellow developer, and a Python whiz, had been silently watching Alex’s growing frustration from the opposite side of the room.
Noticing Alex’s drawn expression, Jordan finally asked, “What’s the issue, Alex? You seem to be wrestling with something.”
With a heavy sigh, Alex leaned back in his chair. “You know how we must implement a uniform interface for all our digital asset classes?
Each asset type must implement ‘render’ and ‘thumbnail’ methods with a specific signature. And it’s becoming a herculean task to ensure that all future asset classes follow this structure. It’s a hotbed for potential errors.”
A light bulb went off in Jordan’s head. “Have you considered using metaclasses?”
Alex’s eyebrows shot up. “Meta… what?”
“Metaclasses,” Jordan clarified with a reassuring smile. “In Python, they offer a powerful way to control class creation. With metaclasses, we can enforce that all digital asset classes implement the necessary methods. It’s a kind of insurance against human error.”
Alex’s interest was piqued. “I’m not very familiar with metaclasses. But I'm all ears if they can make this task easier.”
With a nod of approval from Alex, Jordan delved into an explanation. “Perfect, let’s explore the world of Python metaclasses together. It could be the elegant solution we need.”
And thus, they plunged into a journey of understanding, ready to unravel the mysteries and power of Python’s metaclasses and navigate potential pitfalls.
Introduction to Classes and MetaClasses in Python
Python offers exceptional support for the object-oriented programming paradigm as a high-level, general-purpose programming language. At the heart of this paradigm lies the concept of ‘classes.’
In Python, a class serves as a blueprint for creating objects. An object is a collection of data (variables) and methods (functions) that operate on this data. A class, then, outlines what form these data and methods should take. Here’s a simple example of a class in Python:
class Dog:
def __init__(self, name):
self.name = name
def bark(self):
print(f'{self.name} says woof!')
In the above example, Dog
is a class we can use to create objects (or instances). The __init__
method is a special method we use for initializing class instances, and bark
is a method that all Dog
instances will have.
However, one of the intriguing facts about Python that makes it a dynamically potent language is that classes in Python are themselves objects. This can be subtle at first glance, but it plays a crucial role in Python’s system of classes and objects.
To understand this better, consider that you can manipulate a class just as you would with an object.
This is because when a class is defined (usually with the class
keyword), Python creates an object. The name of the class is then linked to this object, allowing us to interact with it.
We can add attributes, pass them to other functions, store it in data structures, and even instantiate it to create new objects (class instances).
This seemingly paradoxical concept — that classes are objects — leads us to “metaclasses.”
If a class is an object, there must be something that creates this object, right? That something is the metaclass.
In other words, metaclasses are the ‘classes’ that instantiate classes. This may seem unclear initially, but as we delve deeper, we will unravel the workings of metaclasses and their place in Python’s class hierarchy.
This article will thus explore the concept of metaclasses, starting with a clear understanding of classes in Python and their object-like nature and gradually laying a solid foundation for the comprehensive understanding of metaclasses.
Understanding MetaClasses
Having established that classes in Python are objects, it’s crucial to grasp that these objects — classes — must be instances of something. This “something” is a metaclass. In other words, metaclasses can be considered “classes of classes”. They define the rules and behaviors that classes (which are their instances) adhere to.
The type
function as a Metaclass
A key point to understand about metaclasses is that they are typically not used in everyday programming. However, they are an integral part of Python’s internals. The most basic metaclass in Python is type
. When called with one argument, type
returns the type of an object. For example, an integer type is int
, a type (or class) of objects.
print(type(5)) # <class 'int'>
However, type
has another intriguing role when called with three arguments – it behaves as a metaclass, dynamically creating new types (classes). Here is a basic example of type
being used to create a class:
MyClass = type('MyClass', (object,), {'x': 5})
print(MyClass) # <class '__main__.MyClass'>
print(MyClass.x) # 5
In this case, the first argument to type
is the name of the class to create, the second is a tuple containing the base class (or classes) for the new class to inherit from, and the third is a dictionary containing any attributes or methods for the new class
When Does Python Use MetaClasses?
Behind the scenes, Python uses metaclasses to create all new classes, whether or not we realize it. When we define a class using the class
keyword, Python employs a metaclass (by default, this is type
) to create the class. Essentially, metaclasses are responsible for the construction of class objects.
The __metaclass__
attribute
Python provides the __metaclass__
attribute as a way for us to override the default metaclass when defining a new class. By setting __metaclass__
at the class level, we instruct Python to use the specified metaclass instead of type
when creating the class. This can be useful when we want to modify how a class is created, for example, by dynamically adding or changing attributes or methods.
Here’s an example:
class MyClass(metaclass=type):
pass
In this case, MyClass
will still use the type
metaclass because we've explicitly set it, but in reality, we could set metaclass
to any callable accepting the same arguments as type
.
Understanding metaclasses can be challenging, but it is a fascinating journey into Python’s internals, showcasing the language’s flexibility and power.
Creating Your MetaClass
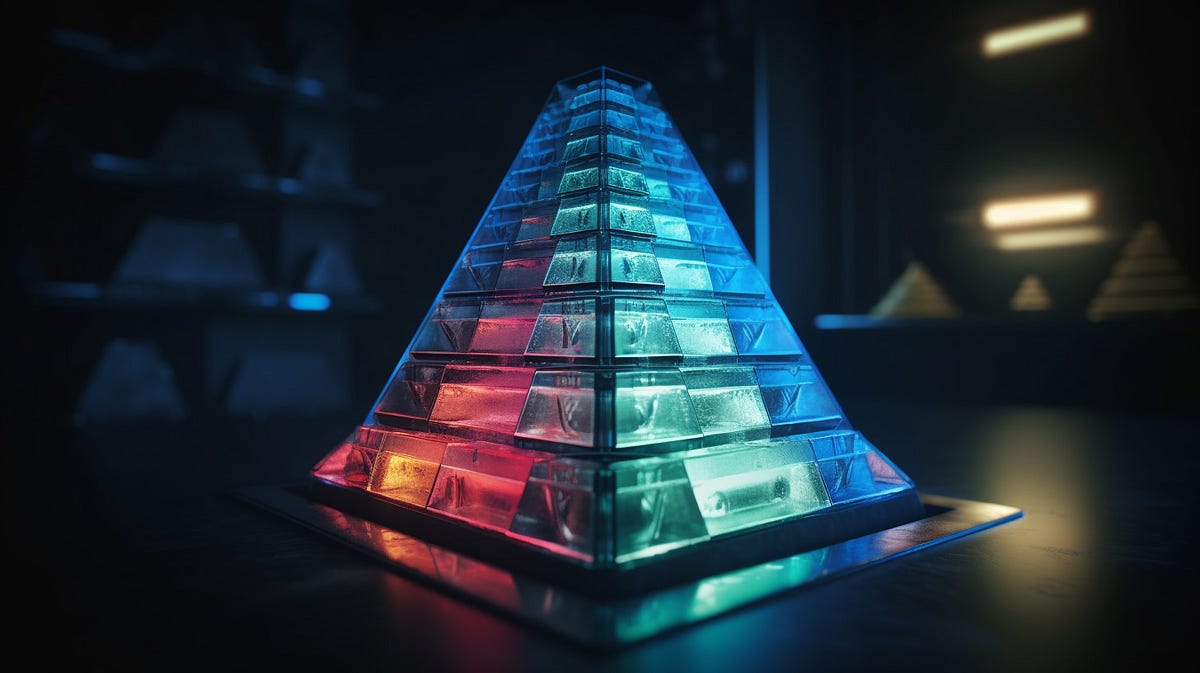
As you’ve learned, all classes in Python are instances of the type
metaclass. If we want to create our metaclass, we can do so by subclassing type
. This way, our metaclass inherits the capabilities of type
, and we can add or override methods as needed.
Here’s a simple example of a custom metaclass:
class Meta(type):
def __new__(cls, name, bases, attrs):
print('Creating class:', name)
return super().__new__(cls, name, bases, attrs)
In this example, Meta
is a metaclass that inherits from type
. It overrides the __new__
method, which is called when creating a new class instance. In the context of metaclasses, this instance is a new class. After printing a message, it calls type
's original __new__
method using the super
function to create the class.
Understanding __new__ and __init__
in the Context of MetaClasses
In Python, __new__
is a static method for creating a new class instance. It's typically overridden when you need to control how new class instances are created, such as when implementing singletons.
In the context of a metaclass, __new__
is responsible for creating new classes. The arguments it receives are the metaclass, the new class's name, the base classes it should inherit from, and a dictionary of attributes and methods.
__init__
, on the other hand, is called after an instance has been created, and it's used to initialize the instance. Like __new__
, you can override __init__
in a metaclass to customize how classes are initialized after they're created. Here's how you can use __init__
in a metaclass:
class Meta(type):
def __init__(cls, name, bases, attrs):
print('Initializing class:', name)
super().__init__(name, bases, attrs)
class MyClass(metaclass=Meta):
pass
In this example, Meta
overrides __init__
to print a message when a class is initialized. When MyClass
is defined using Meta
as its metaclass, it prints "Initializing class: MyClass."
Understanding __new__
and __init__
is key to creating custom metaclasses. They control the class creation and initialization process, allowing you to add custom behaviors to your classes.
Practical Use Cases of MetaClasses
Despite their complexity, metaclasses in Python can be applied in several practical contexts. Here are a few areas where using metaclasses can significantly enhance your programming paradigm.
Automatic Property Creation
Metaclasses can be utilized to create properties automatically in a class. This can be particularly useful when dealing with classes with similar attributes, reducing boilerplate code.
class AutoPropertiesMeta(type):
def __new__(cls, name, bases, attrs):
for attr_name, attr_value in attrs.items():
if isinstance(attr_value, Descriptor):
attrs[attr_name + "_property"] = property(attr_value.getter, attr_value.setter)
return super().__new__(cls, name, bases, attrs)
Validation and Descriptors
Metaclasses can be employed to add automatic validation for class attributes. This can be beneficial in scenarios where certain attribute values must conform to specific rules or constraints.
class ValidatedMeta(type):
def __new__(cls, name, bases, attrs):
# Add attribute validation here
return super().__new__(cls, name, bases, attrs)
Singleton Patterns
A metaclass can be used to implement the Singleton design pattern. This pattern restricts the instantiation of a class to a single instance and is useful in scenarios where a class needs to coordinate actions across a program.
class SingletonMeta(type):
_instances = {}
def __call__(cls, *args, **kwargs):
if cls not in cls._instances:
cls._instances[cls] = super().__call__(*args, **kwargs)
return cls._instances[cls]
ORM and API Development
Object-Relational Mapping (ORM) libraries often use metaclasses to map classes to database tables. This provides an intuitive interface for interacting with the database, as actions on the class correspond to queries on the table.
Similarly, metaclasses can be employed in API development to automatically generate routes based on class methods, significantly simplifying the process of developing web APIs.
Logging and Profiling
Metaclasses can be harnessed to add logging or profiling to methods in a class automatically. This can provide valuable insights into the performance and usage of the methods.
import time
import logging
class LogMeta(type):
def __new__(cls, name, bases, attrs):
for attr_name, attr_value in attrs.items():
if callable(attr_value):
def log(*args, **kwargs):
start = time.time()
result = attr_value(*args, **kwargs)
end = time.time()
logging.info(f'{attr_name} executed in {end-start}s')
return result
attrs[attr_name] = log
return super().__new__(cls, name, bases, attrs)
It’s important to remember that while powerful, metaclasses should be used judiciously, considering their complexity and the impact on code readability and maintainability. They can be a potent tool for addressing advanced programming needs and creating reusable, efficient solutions.
Cautions About MetaClasses
While metaclasses can be a powerful tool in your Python toolkit, it’s crucial to use them carefully. Understanding the potential pitfalls or challenges associated with metaclasses is essential to using them effectively.
Complexity
Firstly, metaclasses can add a considerable layer of complexity to your code. They alter the way Python classes behave, which can confuse other developers unfamiliar with them. Even seasoned Pythonistas can find metaclasses confusing because they change the usual class instantiation process. Therefore, ensuring that your problem requires metaclasses is essential, and a more straightforward approach wouldn’t suffice.
Debugging Difficulties
Given their ability to change class behaviors dynamically, metaclasses can make debugging more challenging. The code that’s written can be quite different from the code executed due to the modifications introduced by the metaclass, making it more difficult to identify the source of a bug.
Compatibility Issues
Metaclasses can also introduce compatibility issues. If a class uses a metaclass, all its subclasses will also use it by default. If a subclass tries to use a different metaclass, Python will raise a TypeError
. This can be problematic when integrating with other code that uses incompatible metaclasses.
Overuse
Lastly, the potential to misuse or overuse metaclasses can lead to ‘metaclass abuse.’ For instance, using a metaclass to add a particular behavior to several classes might be tempting. At the same time, a mixin or a decorator would be more appropriate and more understandable.
For these reasons, many Python developers consider metaclasses an ‘advanced’ feature. They recommend using metaclasses sparingly and judiciously. Before deciding to use a metaclass, exploring more straightforward solutions is always good practice. You should also thoroughly document their use to ensure that future maintainers of your code understand why they were used and how they work.
Remember, “Simple is better than complex,” as per the Zen of Python. Metaclasses, while powerful, should not be the first tool to reach for when faced with a programming problem. Using metaclasses as a last resort is often better when other, more straightforward methods don’t suffice.
Conclusion
In exploring Python’s metaclasses, we’ve taken a deep dive into one of the language’s most advanced features, starting from the fundamentals of classes and gradually traversing the path leading to metaclasses.
We learned that classes in Python are objects created and manipulated like any other. This insight led us to the concept of metaclasses, the ‘classes’ that birth other classes. The type
function, acting as a fundamental metaclass, was discussed, as were the __new__
and __init__
methods that control the class creation and initialization process.
The exploration extended to practical use cases of metaclasses, ranging from automatic property creation and validation, through Singleton patterns and ORM, to logging and profiling. Each instance illuminated the remarkable potential that metaclasses hold if used correctly.
However, the potency of metaclasses also carries a warning. Their complexity, debugging difficulties, compatibility issues, and the risk of overuse advocate for a careful approach.
While they offer a level of dynamism and control few other features can provide, metaclasses should be used sparingly and always as a measured choice rather than a first resort.
Python is a language rich with features designed to make the programming process more intuitive, efficient, and enjoyable. While metaclasses are an example of advanced features, they are only a fraction of Python’s versatile arsenal.
To truly master the language, one must embark on a continuous learning and exploration journey, venturing into every nook and corner Python has to offer.
Finally, remember that Python’s true strength lies not only in its extensive feature set but also in its philosophy — “Readability counts,” “If the implementation is hard to explain, it’s a bad idea,” and “Now is better than never.”
So, continue exploring and learning, but never at the expense of simplicity and readability.
References & Further Reading
Numerous resources provide more detail for those interested in delving deeper into Python’s metaclasses.
Here are some places you can explore to enhance your understanding:
- Python Official Documentation — Classes: Official Python documentation is always a good place to start. It offers an in-depth guide to Python classes.
- Python Official Documentation — The standard type hierarchy: This section of the Python documentation discusses Python’s data model and contains information about metaclasses.
- Python’s Instance, Class, and Static Methods Demystified: This article on Real Python explains Python’s methods well.
- Python 3 Metaprogramming: A video talk by David Beazley at PyData 2013. It provides a comprehensive view of Python metaclasses, their uses, and reasons to avoid them.
- Understanding Python Metaclasses: A blog post by Ionel Cristian Mărieș details metaclasses in Python.
- Stack Overflow — What is a metaclass in Python?: This Stack Overflow thread provides a variety of explanations about what metaclasses are and how they are used in Python.
Remember, while these resources provide a wealth of knowledge, the best way to learn is by doing. Try incorporating metaclasses into your code when appropriate and learn from the experience.
Happy coding and learning!