Unlocking the Power of Python’s Context Managers
Your key to better resource management in Python
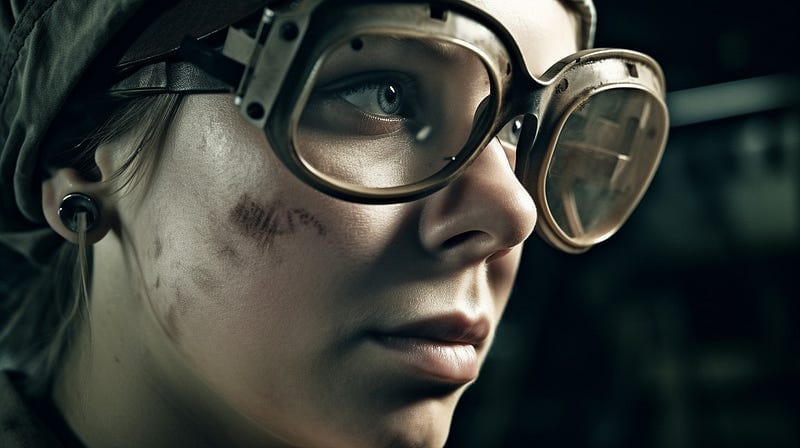
In the bustling hub of Quantum Dynamics Inc., a passionate team of developers was working tirelessly on an ambitious project. They had been meticulously crafting a revolutionary application poised to disrupt the industry.
The code was a beautifully woven tapestry, each line showcasing the team’s dedication and skills. Yet, amidst the intricate lines of Python, a subtle issue was lurking — a memory leak. It was a silent predator, gradually and stealthily consuming system resources.
John, a seasoned developer, and the team’s informal Python guru, had returned from his sabbatical. He was excited to dive back into work and catch up on the project’s progress.
As he delved into the labyrinth of code, he noticed that the application was gradually slowing down. Upon checking the system resources, he found a persistent memory leak.
Looking closely, he traced it back to a block of code that dealt with file operations. The application opened files and read and wrote data, but the files weren’t always closed.
The issue was subtle enough to escape the team’s rigorous code reviews yet significant enough to cause a memory leak. It was like a door left ajar, an invitation to trouble.
John knew this was a classic problem that Python’s context managers were designed to handle. He called for an impromptu team meeting. In the meeting, he discussed the issue while showing the code on the large projector screen. “Python’s context managers,” John began, “are the perfect tool for managing resources like file operations.
They help us ensure that Python disposes of resources after use, even if errors occur. We can use the with
statement to implement this.” He then showed them how the ‘with’ statement could handle file operations.
with open('file.txt', 'r') as f:
contents = f.read()
He explained, “In this code snippet, the with statement creates a context where the file file.txt
is opened. After the operations within the block are completed, the file is automatically closed, even if an error occurs. This is the beauty of context managers.”
The room was filled with a mix of relief and newfound understanding. John had introduced the team to a new Pythonic way of handling resource management, and they were excited to put this knowledge into practice.
From that day on, Python’s context managers became integral to their coding toolkit, saving them from many potential headaches and making their code more elegant and efficient.
Table of contents
· Managing Resources in Python
∘ Handling resource management
· Understanding Context Managers
∘ The Importance of Context Managers
· Creating Custom Context Managers
∘ Custom logger context manager
∘ Custom timer context manager
∘ Temporary directory context manager
· The @contextlib.contextmanager Decorator
∘ Advantages
∘ Disadvantages
· Nested Context Managers
∘ Implications and Benefits
· Real-world Applications of Context Managers
∘ File Management
∘ Database Connections
∘ Locks in Multithreading
∘ Temporary state changes
∘ Testing and Mocking
· Conclusion
Managing Resources in Python
As the introduction shows, one common challenge in programming is the efficient management of external resources such as files, locks, and network connections.
A program could retain these resources indefinitely, even after their utility has ended. This is called a memory leak. The available memory shrinks whenever you create and open a new resource instance without closing an existing one.
Dealing with resources often poses a tricky problem, necessitating setup and teardown phases. The last step requires the execution of specific cleanup actions, such as closing a file, releasing a lock, or terminating a network connection.
If a developer overlooks these cleanup actions, your application will keep the resource alive. This compromises valuable system resources like memory and network bandwidth.
Several instances can illustrate this problem. For example, a program could continuously create new database connections without releasing them. Consequently, the database backend might stop accepting new connections. A database admin needs to intervene and terminate these connections.
Another common issue arises when dealing with files. Writing text to files is a buffered operation, implying that invoking .write()
on a file doesn’t immediately write text to the physical file but to a temporary buffer. Sometimes, if the buffer isn’t full and developers forget to call .close()
, data could be lost forever.
Your application might encounter problems or unexpected situations that skip over the code meant to free up the resource.”
Below is an example that uses open()
to write text to a file.
file = open("demo.txt", "w")
file.write("Possible, exception might occur!")
file.close()
In this code snippet, we open a file, write a message, and close it. But, this implementation has a hidden pitfall. It does not guarantee Python will close the file if the program throws an exception when executing the write() method.
If an error occurs in this critical section, Python will bypass the close() method leaving the file open. This situation can lead to a file descriptor leak in your program, where a system resource is consumed but not released.
Handling resource management
To handle resource management in Python, you typically have two primary strategies at your disposal:
- Using a
try…finally
Construct: This traditional approach allows you to provide setup and teardown code to manage any resource type. However, while flexible, it can be somewhat verbose and prone to human errors. There’s always the risk of forgetting to include necessary cleanup actions, which could lead to resource leaks. - Using a
with
Construct: This alternative method offers a more straightforward way to encapsulate and reuse setup and teardown code. The limitation here is that thewith
statement works exclusively with context managers. However, when used appropriately, it can simplify your code and ensure that resources are correctly managed, even in the face of unexpected exceptions.
In the upcoming sections, we will dive deeper into the with
construct. This will equip you with the knowledge and tools to effectively manage resources in your Python code using context managers, thus preventing those pesky resource leaks.
Understanding Context Managers
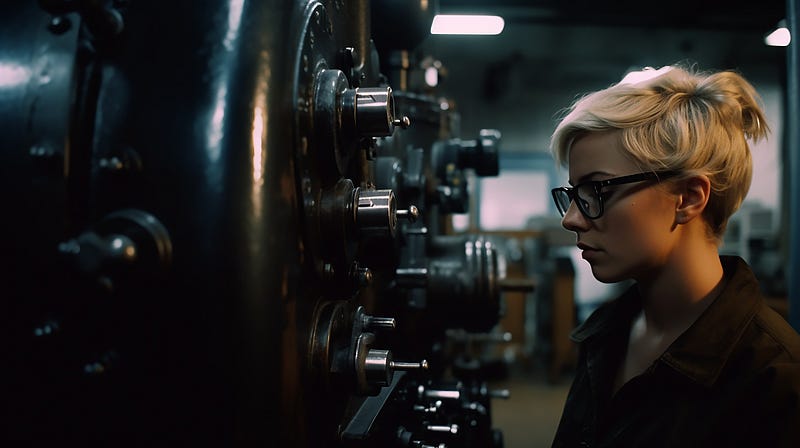
A context manager in Python is an object that defines methods to be used in conjunction with the with
keyword.
The concept of context managers comes from a programming paradigm known as Resource Acquisition Is Initialization (RAII), where acquiring a resource is coupled with initializing an object.
The two key methods a context manager defines are __enter__()
and __exit__()
.
The __enter__()
method is executed when the with
block is entered, and __exit__()
is called when the with
block is exited, whether the block is exited normally or due to an exception.
This is the magic behind context managers - they ensure that certain cleanup operations are always performed, regardless of whether an operation within the block was successful or raised an error.
Here’s an example of a with
block using a file object, which is a built-in context manager in Python:
with open("demo.txt", "w") as file:
file.write("Hello, World!")
The Importance of Context Managers
Context managers play a crucial role in managing resources by providing a mechanism for automatic setup and teardown. They make code cleaner, more readable, and more resilient to errors.
By using context managers, you can ensure that resources like files or network connections are correctly cleaned up after use, even in the face of unexpected exceptions. This helps prevent resource leaks, which can slow down your program and consume valuable system resources.
Moreover, context managers help encapsulate the logic of setup and teardown within the objects that use these resources, making your code more modular and easier to maintain.
By handling these details internally, context managers let you focus on the core logic of your program, making your code safer and more efficient.
Creating Custom Context Managers
While Python provides several built-in context managers like files and locks, there may be times when you need to create a custom context manager for specific tasks.
Python allows you to define your own context managers by creating a class and implementing the __enter__
and __exit__
methods.
The __enter__
method is where you'll include any setup code that your context requires. This method is automatically called when execution enters the scope of the with
statement. The __exit__
method, on the other hand, contains the teardown code.
This method is called when execution leaves the scope of the with
statement, even if an exception was raised within the block.
Custom logger context manager
Here’s an example of a custom context manager that manages a simple logging operation:
class CustomLogger:
def __enter__(self):
self.log_file = open("log.txt", "w")
return self
def write_log(self, log_message):
self.log_file.write(log_message + "\n")
def __exit__(self, exc_type, exc_val, exc_tb):
if exc_type:
self.write_log(f"An exception of type {exc_type} occurred.")
self.log_file.close()
In this example, the CustomLogger
class is a context manager that opens a log file when entering the context and closes the file when exiting. You can write messages to the log file using the write_log
method.
If an exception occurs within the with
block, it logs the exception type before closing the file.
Here’s how you’d use this custom context manager:
with CustomLogger() as logger:
logger.write_log("This is a log message.")
# any other code
In this code, the __enter__
method is called, opening the log file. The write_log
method is used to write a message to the file. Finally, the __exit__
method is called when the with
block is exited, closing the log file. If an exception had occurred within the with
block, the exception type would have been logged before closing the file.
This custom context manager ensures that the log file is always closed properly, even if an error occurs, and provides a simple, reusable way to manage logging operations.
Custom timer context manager
Here is another example of a context manager. This context manager can measure the execution time of a block of code. This can be useful for performance testing.
In this example, the Timer
class is our custom context manager. When we enter the with
block, the __enter__
method is called and it records the current time.
When we exit the with
block, the __exit__
method is called, it re-records the current time, and then prints the difference between the end and start times. This difference is how long it took to execute the block of code inside the with
statement.
Even if an exception occurs within the with
block, the __exit__
method will still be called, ensuring we always get a timing for our code block. This is a simple example of how you can create a custom context manager to handle a common task clean and reusable way.
import time
class Timer:
def __enter__(self):
self.start = time.time()
return self
def __exit__(self, exc_type, exc_val, exc_tb):
self.end = time.time()
print(f"Block took {self.end - self.start} seconds to execute.")
# Usage:
with Timer():
for i in range(1000000):
pass
Temporary directory context manager
Let’s create a context manager for a temporary directory. This can be useful when you need a temporary workspace for file operations which should be cleaned up after use.
In this example, TempDirectory
is our custom context manager. When we enter the with
block, the __enter__
method is called, creating a new temporary directory. The path to this directory is returned and assigned to the variable temp_dir
.
You can then use temp_dir
within the with
block to create files and directories for temporary use.
When we exit the with
block, the __exit__
method removes the temporary directory and all its contents, even if an error occurred.
This is a valuable way to ensure that temporary files and directories are always cleaned up properly, preventing disk space leaks in your program.
import os
import tempfile
import shutil
class TempDirectory:
def __enter__(self):
self.temp_dir = tempfile.mkdtemp()
return self.temp_dir # This value will be assigned to the variable after 'as'
def __exit__(self, exc_type, exc_val, exc_tb):
shutil.rmtree(self.temp_dir)
# Usage:
with TempDirectory() as temp_dir:
# Inside this block, temp_dir is a path to a temporary directory
print(f"Doing work in {temp_dir}")
# You can create files and directories inside temp_dir, and they will be cleaned up automatically
The @contextlib.contextmanager Decorator
While creating a class with __enter__
and __exit__
methods is a straightforward way to create a context manager, Python provides a more elegant way to create one using the contextlib
module's contextmanager
decorator.
The @contextlib.contextmanager
decorator is a simpler and more Pythonic way to create context managers without needing a full class. It allows you to create a context manager using a single generator function instead of a class. The decorated function should yield exactly once. The code before the yield
statement is considered the __enter__
method, and the code after is the __exit__
method.
Here’s the Custom timer context manager example implemented using @contextlib.contextmanager
. In this example, the timer
function is a context manager that records the start time, yields control back to the with
block, and then, once the block is exited, records the end time and prints the elapsed time.
This is functionally equivalent to the class-based Timer
context manager we defined earlier but is achieved with less code and a simpler structure thanks to the @contextlib.contextmanager
decorator.
import time
import contextlib
@contextlib.contextmanager
def timer():
start = time.time()
yield
end = time.time()
print(f"Block took {end - start} seconds to execute.")
# Usage:
with timer():
for _ in range(1000000):
pass
When juxtaposing the implementation of a context manager through a class-based approach versus the usage of the @contextlib.contextmanager
decorator, each method presents its own advantages and disadvantages.
Advantages
- Simplicity: Using
@contextlib.contextmanager
allows you to create a context manager with less boilerplate code than defining a class with__enter__
and__exit__
methods. - Readability: Since it uses a single function, the
@contextlib.contextmanager
approach can be more readable and intuitive, especially for Python developers familiar with decorators and generators.
Disadvantages
- State Management: For more complex context managers that need to maintain and manage their own state, using a class can be a more suitable choice. The
@contextlib.contextmanager
approach may not be as clean or intuitive for these scenarios. - Error Handling: The
__exit__
method in a class-based context manager can suppress exceptions by returning a truthy value. This is not directly possible in a context manager created using@contextlib.contextmanager
. Instead, you'll need to catch and handle exceptions within the generator function.
The @contextlib.contextmanager
decorator is a powerful tool for creating simple and readable context managers. For more complex use cases, a class-based approach might be more suitable.
Nested Context Managers
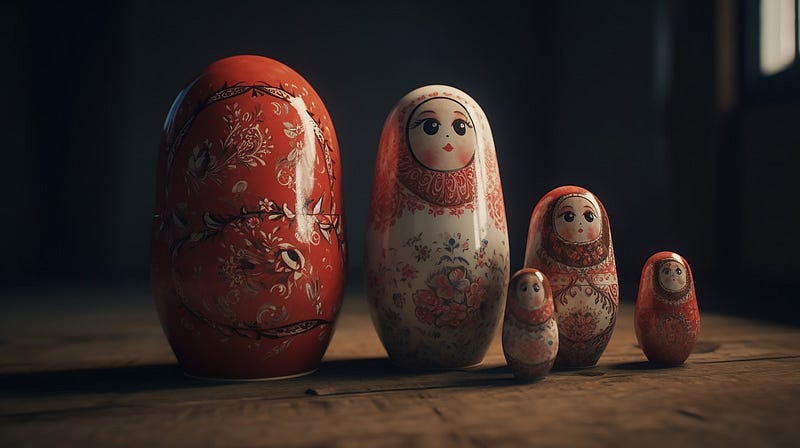
Python allows the use of multiple context managers in a single with
statement. This will enable you to set up and tear down multiple resources neat and organized, and ensures that all resources are properly cleaned up, even in the case of an error. This is referred to as "nesting" context managers.
Here’s an example of how to use multiple context managers in a single with
statement. In this example, we’re opening two files for writing. The with
statement ensures that both files are properly closed, even if an error occurs while writing to the files.
with open('hello.txt', 'w') as hello_file, \
open('world.txt', 'w') as world_file:
hello_file.write('Hello, ')
world_file.write('World!')
Implications and Benefits
Nested context managers have several implications and benefits:
- Simplicity: Using multiple context managers in a single
with
statement simplifies your code and makes it easier to read. - Resource Management: It ensures that Python manages all resources. In the above example, even if writing to
hello_file
fails,world_file
will still be adequately closed. - Error Isolation: If an exception occurs while managing one resource, the other resources are unaffected. In the above example, an error writing to
hello_file
does not preventworld_file
from being written to or closed. - Code Organization: Nesting context managers help to logically group code that manages related resources, which improves code readability and maintainability.
By understanding and utilizing nested context managers, you can write more robust and cleaner Python code, especially when dealing with multiple resources that all need proper setup and teardown.
Real-world Applications of Context Managers
Context managers are potent tools and can be found in various real-world programming scenarios, where they simplify resource management and make the code cleaner and more readable. Here are some of the key applications.
File Management
As we have seen in the previous examples, file handling is a common use case for context managers. They ensure files are properly closed after operations, even if an error occurs.
with open('file.txt', 'r') as f:
contents = f.read()
# At this point, f is guaranteed to be closed, whether or not an exception occurred
Database Connections
Similar to file handling, context managers are useful for managing database connections. They ensure that connections are properly closed or returned to the connection pool, even if an error occurs during an operation.
from contextlib import closing
from sqlite3 import connect
with closing(connect('movies.db')) as conn:
cursor = conn.cursor()
cursor.execute('SELECT * FROM movies')
# At this point, conn is guaranteed to be closed
Locks in Multithreading
In multithreading, we often use locks to prevent multiple threads from accessing shared resources simultaneously. Context managers are a great way to handle acquiring and releasing locks.
from threading import Lock
lock = Lock()
with lock:
# Perform some operations on shared resource
# At this point, lock is guaranteed to be released
Temporary state changes
Sometimes, you want to temporarily change a state, do something with that state, and then change it back, whether the operation was successful or not. Context managers are perfect for this.
import numpy as np
with np.errstate(divide='ignore'):
# Perform some operation that could result in division by zero
# At this point, the original error state is restored
Testing and Mocking
In the realm of testing, we often want to mock certain behaviors or variables during the execution of a test, and then ensure those changes are undone after the test. Context managers are excellent for this purpose.
Consider the unittest.mock.patch
object from Python's standard library, which is a context manager. It allows you to replace parts of your system under test and assert how they were used.
In this example, the patch
context manager temporarily replaces my_module.my_function
with a mock. Within the with
block, you can perform tests and assert how my_module.my_function
is used. Once the block is exited, my_module.my_function
is restored to its original state, ready for the next test.
from unittest.mock import patch
with patch('my_module.my_function') as mock_my_function:
# my_module.my_function is temporarily replaced by a mock for the duration of this block
# Perform some tests that might call my_module.my_function
mock_my_function.assert_called_once()
# After the block, my_module.my_function is the original function again
Conclusion
Python context managers are powerful tools that take the complexity out of resource management. By automating the setup and teardown processes, they ensure resources are correctly and efficiently handled, helping to avoid common problems like memory leaks and system slowdowns.
Our journey in this article has taken us from understanding the importance of context managers to creating custom context managers and even leveraging Python’s @contextlib.contextmanager
decorator for simpler implementation.
We have seen how to use multiple context managers in a nested fashion and explored real-world applications of context managers in file management and database connections.
Though fictional, the example of Quantum Dynamics Inc. illustrates a common problem many developers face in their daily work. By applying the principles and practices of context managers, as demonstrated by the Python guru John, developers can overcome similar challenges and craft more efficient, robust, and elegant code.
Context managers are more than a feature of Python; they embody a mindset of resource consciousness and efficiency. Whether you’re a seasoned developer or a beginner, properly using context managers is a valuable addition to your Python skillset.
Remember, resource leaks can be silent predators, but with Python’s context managers, you’re well-equipped to keep them at bay.
Happy coding!