Navigating Python’s Exception Landscape — A Comprehensive Guide From Basics to Best Practices
Decoding Python’s exception handling: Tracebacks, multiple except blocks, the ‘else’ and ‘finally’ blocks, ‘raise’ statements, assertions…
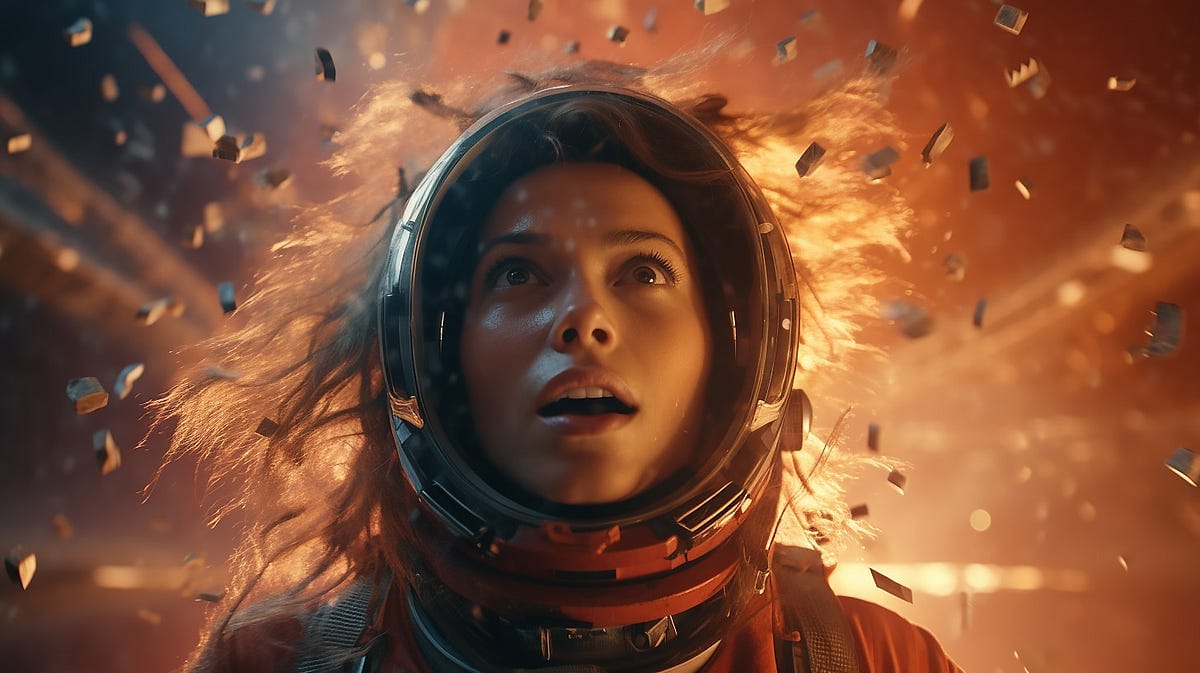
Decoding Python’s exception handling: Tracebacks, multiple except blocks, the ‘else’ and ‘finally’ blocks, ‘raise’ statements, assertions, and industry-standard practices
Embarking on a Python adventure, you’ll inevitably traverse the rocky terrain of errors and issues. Be it a missing file, a failed network or database connection, or encountering invalid input, your journey is bound to involve navigating such obstacles.
The beacon that can guide you through these challenging circumstances is the Python ‘raise’ statement — an effective signal flare that alerts the user of the occurrence of an error.
Diving into the world of the ‘raise’ statement enables you to manage errors and exceptional situations in your code adeptly. With this skill, you can craft more resilient programs and elevate the quality of your code.
In this article, we’re setting out on an exploration to discover how to:
- Handling exceptions in Python
- Invoke exceptions in Python using the ‘raise’ statement
- Strategize on which exceptions to invoke and identify the appropriate circumstances to do so
- Investigate common scenarios where raising exceptions in Python is necessary
- Implement best practices for exception handling in your Python code
So, fasten your coding seatbelt and prepare for an in-depth expedition into the world of exceptions in Python.
Table of contents
· Understanding Exceptions
∘ Traceback
· Basic Exception Handling
∘ Using Multiple Except Blocks
∘ The Else Block
∘ The Finally Block
· Leveraging Python’s raise statement
· Understanding Assertions in Python
· Navigating Exceptions in Python: Best Practices
· Wrapping Up
Understanding Exceptions
An exception is thrown in Python whenever a mistake or an unexpected scenario crops up. These exceptions come in different flavors, each representing a unique error.
Some typical ones include:
TypeError
: This occurs when an operation or function is applied to an object of an inappropriate type.ValueError
: Raised when a built-in operation or function receives an argument with the correct type but inappropriate value.IndexError
: Encountered when you attempt to reach a non-existing index in a list.FileNotFoundError
: This happens when a file or directory is requested but cannot be found.
An entire catalog of built-in exceptions is available in the Python documentation that you can consult for a more comprehensive understanding.
To illustrate, imagine you’re trying to retrieve an item from a list
using an index that doesn’t exist.
In Python, list indices start from 0 and end at n-1
(where n
is the total number of items in the list). So, in this answers
list, the indices range from 0 to 3, corresponding to four elements in total.
Imagine trying to print the element at the 4th index.
answers = [
"bush",
"fish",
"grass",
"bloom"
]
print(answers[4])
Oops! Our answers
list doesn't have an index 4. It stops at 3. Consequently, Python will throw an IndexError
exception, signaling that the index you're trying to access exceeds the actual list boundary. It's Python's saying, "Hey, you're trying to access a list element that simply isn't there!"
╰─ python3 list_index_error.py
Traceback (most recent call last):
File "/Users/kalkman/Projects/st/python-exception/list_index_error.py", line 8, in <module>
print(answers[4])
IndexError: list index out of range
╭
Traceback
Each exception that gets raised in Python comes with a companion known as a traceback — it’s also referred to by several other monikers such as a stack trace, stack traceback, or simply backtrace. Think of a traceback as a detective’s report, carefully detailing the chain of events or sequence of operations leading up to the moment the exception occurred.
In Python, a traceback typically begins with the header Traceback (most recent call last)
. It might seem cryptic at first, but it simply means that the traceback will list the series of calls made in reverse order, starting from the most recent function or method that was called before the exception was encountered and tracing back through the sequence of calls that led to it.
Following this header, you’ll find the actual sequence of calls made — the so-called “call stack”. Lastly, the report concludes with the name of the raised exception and a helpful error message providing more insight into why the exception occurred. This traceback mechanism provides a powerful tool to diagnose and debug your Python programs.
Exceptions are like speed bumps on your program’s path, and if left unattended, they can bring your program to a screeching halt.
However, Python provides a safety net to prevent such abrupt terminations — the try...except
block. This protective measure allows your program to continue running, even when encountering an exception, by implementing proper exception handling.
Basic Exception Handling
To truly excel at Python programming, you must predict and handle exceptions. Failure to do so can result in your program terminating unexpectedly.
In such scenarios, Python lends a helping hand by generating a traceback — a detailed account of the sequence of events leading to the error. Interestingly, intentionally allowing a program to fail sometimes unveils hidden exceptions.
Take our earlier list indexing example; attempting to access a non-existent index inevitably raises an IndexError
.
Here, Python's try...except
block comes to the rescue—it's a protective measure that catches and handles exceptions. Consider the following:
answers = [
"bush",
"fish",
"grass",
"bloom"
]
try:
print(answers[4])
except IndexError:
print("IndexError: there is no answer in your list with index 4")
Running this script now results in a handled IndexError
, and our custom error message gets printed:
╰─ python3 list_index_error_handle.py
IndexError: there is no answer in your list with index 4
If an exception is thrown within a function and isn’t handled right there, it percolates up through the layers of the program — escalating from the point of function invocation up to the main program. Without any exception handler, the program stops, providing an exception traceback for debugging.
Remember, exceptions are the lifeblood of Python. They’re ubiquitously used throughout the standard library and can arise in many circumstances. As stated in the Python documentation:
Exceptions are a means of breaking out of the normal flow of control of a code block in order to handle errors or other exceptional conditions. An exception is raised at the point where the error is detected; it may be handled by the surrounding code block or by any code block that directly or indirectly invoked the code block where the error occurred.
Using Multiple Except Blocks
Python allows using multiple except
blocks to handle different types of exceptions. This capability helps create more robust error-handling mechanisms, allowing specific reactions to different error scenarios.
Let’s revisit our list example and enhance it to handle a broader range of exceptions:
answers = [
"bush",
"fish",
"grass",
"bloom"
]
try:
print(answers[5])
except IndexError:
print("IndexError: There is no answer in your list at index 5!")
except Exception as e:
print("An unexpected error occurred:", str(e))
Here, the first except
block handles the IndexError
, while the second handles any other type of exception.
The Else Block
In Python, you can use an else
block alongside try
and except
. The else
block springs into action when the try
block sails through without encountering any exceptions.
Here’s an example to illustrate this concept:
try:
print("Hello, Medium!")
except Exception as e:
print("An error occurred:", str(e))
else:
print("Smooth sailing - no errors encountered!")
In this scenario, the output will be “Hello, Medium!” and “Smooth sailing — no errors encountered!” as the try block proceeds without a hiccup, triggering the other block. This else
block provides an excellent opportunity to include code that should only be executed if no exceptions were raised in the try
block.
The Finally Block
The finally
block is used for cleaning up resources or executing statements that must occur whether an exception is raised.
try:
print("Hello, Medium!")
except Exception as e:
print("An error occurred:", str(e))
finally:
print("This statement is always executed.")
“Hello, Medium!” and “This statement is always executed.” will be printed.
Leveraging Python’s raise
statement
In Python, exceptions are not merely obstacles to sidestep; they can be powerful tools. Python grants us the ability to raise both built-in and custom exceptions. Initiating these exceptions using the raise
statement yields the same outcome as Python's built-in exceptions: a traceback report and a potentially halted program unless the exception is promptly managed.
It’s worth noting a slight distinction in terminology: in Python, we raise exceptions, while in languages like C++ or Java, exceptions are thrown.
The raise
statement syntax is generally as follows:
raise [expression [from another_expression]]
In the context of an active exception, a raise
keyword without an argument will re-raise the current exception. This must be executed within except
code blocks with an active exception. If used outside of this context, a RuntimeError
exception is raised.
This basic form of the raise
statement becomes especially useful when you must perform some actions after catching an exception before re-raising the original exception.
The object in the raise
statement should be an instance (or a class) derived from BaseException
, the parent class for all built-in exceptions. If the object is a class, Python automatically instantiates it for you.
The from
clause in the raise
statement is optional and allows you to chain exceptions together, linking one exception to another.
Consider this illustration:
raise Exception("An error occurred.")
Here, you’re raising an instance of Exception
for illustrative purposes, though generally, it's not a recommended practice. User-defined exceptions should be derived from this class or other built-in exceptions.
In Python, error messages for exceptions typically start with a lowercase letter and don’t end with a period. Consider the following examples:
42 / 0 # Results in ZeroDivisionError: division by zero
[][0] # Results in IndexError: list index out of range
Exception classes like Exception
can take multiple positional arguments or a tuple of arguments. Typically, exceptions are instantiated with a single string argument as an error message. Still, they can also be instantiated with multiple arguments to provide additional information about the cause of the error.
The .args
attribute gives you access to all the arguments passed to the Exception constructor, as shown below:
error = Exception("An error occurred", "unexpected value", 42)
print(error.args) # Outputs: ('An error occurred', 'unexpected value', 42)
print(error.args[1]) # Outputs: 'unexpected value'
Having grasped the basics of raising exceptions in Python, the next step is to discern which exceptions are appropriate for specific situations. This critical decision shapes the error-handling strategy and improves the robustness of your code.
Understanding Assertions in Python
Python provides the raise
statement and the assert
statement for triggering exceptions. However, the assert
statement has a distinct purpose and is designed only to raise one specific type of exception, the AssertionError.
The assert
statement is a practical tool for debugging and testing in Python. It facilitates sanity checks, also known as assertions, that allow you to verify the validity of certain assumptions in your code. If any assertion proves false, the assert
statement triggers an AssertionError
exception. Encountering this error often indicates the presence of a bug in your code.
Remember, the assert
statement is unsuitable for handling user input or other input errors. The rationale behind this is that assertions can be—and often are—disabled in production code. Consequently, they are best used as debugging and testing tools during development.
The syntax for the assert
statement in Python is as follows:
assert expression[, assertion_message]
Here, expression
can be any valid Python expression or object you wish to test for truthiness. If expression
is evaluated as false, an AssertionError
is raised. The optional assertion_message
parameter provides additional context for debugging and testing, allowing for an error message that describes the problem the statement aims to identify.
Consider the following example demonstrating the use of an assert
statement with a descriptive error message:
age = -10
assert age >= 0, f"Expected age to be at least 0, got {age}"
Upon executing this code, an AssertionError
is raised as the assertion is false, with the provided message clearly indicating the issue causing the failure. As a reminder, validating input should not rely on the assert
statement, as it can be turned off in production code and thus bypass the intended validation.
Lastly, it’s important to highlight that you should not explicitly raise the AssertionError
exception using a raise
statement. The occurrence of this exception should be a result of a failing assertion during the testing and debugging process of your code development.
Navigating Exceptions in Python: Best Practices
As you journey through the terrain of Python exception handling, certain practices can make your task significantly easier and more efficient. Here’s a roundup of some of these key practices:
- Specificity Over Generality: Always opt for the most specific exception that aligns with your needs. This strategy facilitates easier identification and resolution of issues.
- Explicit Error Messaging: Ensure all exceptions are accompanied by descriptive and clear error messages. This provides valuable context to anyone debugging the code.
- Prioritize Built-in Exceptions: Search for a suitable built-in exception that aligns with your code’s error before crafting your custom exception. Leveraging built-in exceptions promotes consistency with the larger Python ecosystem and enables other developers to comprehend and work with your code more easily.
- Avoid
AssertionError
Misuse: Refrain from raising theAssertionError
in your code. This exception is reserved for theassert
statement and unsuitable for other contexts. - Prompt Exception Raising: Introduce error checks and detect exceptional situations as early as possible in your code. This increases code efficiency by avoiding unnecessary computations that could be discarded by a delayed error check. This aligns with the fail-fast design principle.
- Document Your Exceptions: Meticulously list and explain all the exceptions a specific code could raise within your code’s documentation. This aids other developers by clarifying the exceptions they should anticipate and providing guidance on appropriate handling strategies.
Wrapping Up
Mastering the art of handling exceptions is fundamental to crafting sturdy and reliable Python code. Exception handling equips your program with the capacity to tackle unexpected errors gracefully and persist in its execution.
This guide endeavored to present a thorough exploration of exceptions in Python, encompassing their creation, handling, and best practices.
The knowledge acquired from understanding these concepts will significantly enhance the robustness and dependability of your Python applications.
By embracing these principles, you’re on the path toward creating more fault-tolerant code that can withstand unexpected scenarios.
It’s time to apply these newfound skills to your Python coding. Remember, practice is the key to mastering any concept, and exception handling is no different.
Continue exploring, keep coding, and most importantly, enjoy the journey.
Happy coding!