Mastering Global Variables — Enhancing Python Functions With Global Scope
A guide to understanding, utilizing, and creating global variables in your Python programs
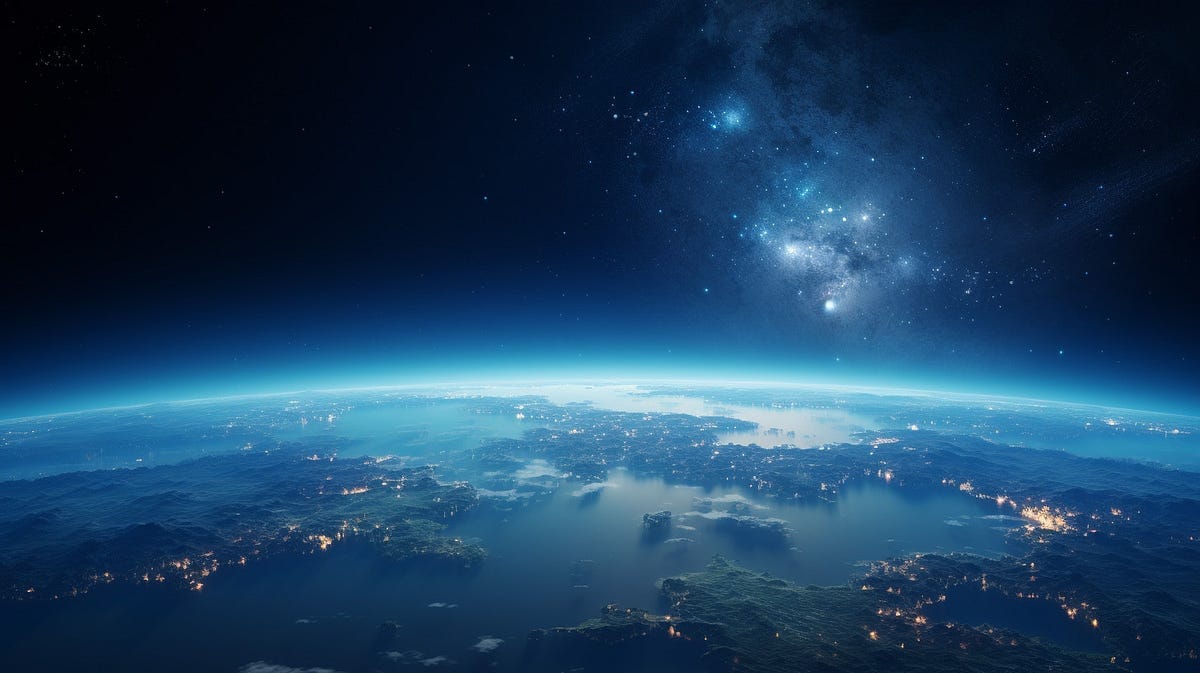
“Good morning, Aaron!” A cheerful voice broke through the office’s usual hum of keystrokes and coffee sips. It was Emily, a bright and enthusiastic intern who recently joined the development team.
“Morning, Emily. How can I assist you today?” Aaron asked, looking up from his dual-monitor setup, welcoming the interruption from his intricate Python code.
“I’ve been trying to fix a piece of code, but it’s been giving me trouble all morning. It’s about changing a global variable inside a function. It just doesn’t work as I expect,” Emily admitted, her usual bright demeanor slightly dimmed by the struggle.
“Oh, you’ve encountered a common pitfall. Let’s take a look,” Aaron offered, clearing a space on his desk for Emily’s laptop.
Emily pulled up her Python script. She had a counter, a global variable, that she was incrementally updating inside a function. But every time she printed the counter outside the function, it wasn’t reflecting the updated value.
“Ah, I see the issue. You’re trying to modify a global variable inside the function,” Aaron pointed out, his years of Python experience shining through.
“But isn’t it possible? If a variable is declared globally, isn’t it accessible everywhere?” Emily queried, her brows furrowed in confusion.
“Yes, you’re right,” Aaron agreed, “A global variable can be accessed from anywhere in your code. However, to modify it inside a function, you need to declare it as global within that function scope explicitly.”
Emily’s expression changed from confusion to curiosity. “Why is that, though?”
Aaron leaned back in his chair, prepared to explain a core aspect of Python, “You see, when you create a variable inside a function, it’s local by default. This means the variable can only be accessed and modified within that function. But when you use the global
keyword before the variable inside the function, you're telling Python that you want to use the globally defined variable, not create a new local one."
“So, when I tried to change the counter inside the function without the global
keyword, Python looked at it as a local variable?" Emily asked, her eyes beginning to light up with understanding.
“Exactly,” Aaron confirmed, his fingers dancing on the keyboard, quickly modifying Emily’s code to include the global
keyword. The script worked flawlessly, updating and printing the global counter as Emily originally intended.
“Got it! That makes a lot of sense. Python variable scope can be trickier than it seems!” Emily said, a satisfied grin returning to her face. She thanked Aaron, her mind already churning on applying her newfound knowledge to the rest of her code.
As Emily walked away, Aaron mused on the importance of understanding variable scope in Python. It might seem complex at first glance, but with a solid grasp of local, global, and the ‘LEGB’ rule, one could navigate Python programming much more proficiently.
Table of contents
· Understanding variable scope in Python
∘ Defining local and global scope
∘ The ‘LEGB’ Rule (Local, Enclosing, Global, Built-in)
∘ Examples illustrating variable scope
· The role of global variables in Python
∘ When and why to use global variables
∘ Potential problems and considerations with global variables
· Using global variables in Python functions
∘ The syntax for accessing global variables
∘ Examples of using global variables in functions
· Creating and modifying global variables within functions
∘ How to declare global variables within a function
∘ Modifying global variables inside a function
· Best practices and alternatives to global variables
∘ When to avoid using global variables
∘ Alternatives to global variables
∘ Example of refactoring a function that uses global variables
· Global variables and multi-threading
∘ A brief overview of multi-threading in Python
∘ Potential problems with global variables and threads
∘ Strategies for dealing with these problems
· Conclusion
Understanding variable scope in Python
Before we dive into manipulating and creating global variables within functions, it’s crucial to understand the concept of variable scope in Python. Variable scope can be considered the region in the code where a variable is visible and can be accessed. In Python, variables are local or global scope, determined by where they are declared.
Defining local and global scope
A local variable is declared within a function and can only be used in the scope of that function. Once the function finishes execution, the local variable is destroyed, and trying to access it outside the function will raise an error.
A global variable, on the other hand, is declared outside of all functions and is accessible throughout the entire program. Global variables hold their value throughout the program's life and can be accessed and modified by any function unless it declares a local variable with the same name.
The ‘LEGB’ Rule (Local, Enclosing, Global, Built-in)
When a variable is referenced in Python, the interpreter follows the LEGB rule to find where it was defined. The letters in LEGB stand for Local, Enclosing, Global, and Built-in.
- Local: Variables defined within a function
- Enclosing: Variables in the local scope of any enclosing functions from inner to outer
- Global: Variables defined at the top level of a module or explicitly declared global using the ‘global’ keyword
- Built-in: Predefined variables in Python, such as special attributes of data types and functions
The Python interpreter starts searching at the innermost scope and proceeds outwards. It stops as soon as it finds the first match.
Examples illustrating variable scope
Let’s consider a simple Python program to demonstrate variable scope:
# Global variable
x = 10
def local_example():
# Local variable
y = 5
print("Local variable y = ", y)
local_example()
print("Global variable x = ", x)
In the program above, x
is a global variable, and y
is a local variable inside the function local_example
. When you run this code, it prints out the local variable y
inside the function, and it also prints out the global variable x
outside the function.
If you try to print y
outside the function, it will raise an error, since y
is not in the global scope.
print("Local variable y = ", y) # This will raise a NameError
Understanding the distinction between local and global scope, and how Python’s LEGB rule operates is the foundation for effectively using and creating global variables in your Python programs. In the next sections, we’ll delve deeper into how to put these concepts into practice.
The role of global variables in Python
As you journey deeper into Python programming, you’ll encounter a variety of strategies for managing data within your applications. Among these, using global variables is a powerful tool and a source of potential pitfalls. Understanding when, why, and how to use global variables effectively is a key skill in Python programming.
When and why to use global variables
Global variables are variables that are defined outside of any function and are accessible throughout your entire program. They hold their value throughout the life of your program. They can be accessed and modified by any function, which makes them particularly useful for storing data that needs to be shared across multiple functions.
Here are a few instances when using global variables can be appropriate:
- Constants: Global variables are excellent for defining constants that need to be accessed by multiple functions. For instance, a global variable could be used to store the version number of your software or common configuration settings.
- Shared Resources: If multiple functions in your program need to interact with the same resource, such as a database connection or a file, it may be practical to use a global variable to store that resource.
- Stateful Programs: In some cases, your program might need to maintain some “state” that persists across function calls. A global variable can be used to store this state information.
Potential problems and considerations with global variables
While global variables can be handy, they should be used sparingly and thoughtfully. Overusing or misusing global variables can lead to difficult code to understand, debug, and maintain. Below are a few potential pitfalls to keep in mind:
- Namespace Clashes: Global variables are accessible throughout your program, increasing the risk of naming conflicts. This can lead to bugs that are hard to trace and fix.
- Modifiability: Since any function can access and modify global variables, they can easily be changed inadvertently. This makes predicting the state of a global variable at any given time challenging.
- Testing and Debugging Difficulties: Code heavily relying on global variables can be difficult to test and debug since global variables' functions carry hidden dependencies.
- Concurrency Issues: In multi-threaded programs, global variables can lead to data inconsistencies if they are modified by different threads simultaneously.
Limiting your use of global variables is a good rule of thumb in light of these considerations. Instead, prefer function parameters and return values, which make dependencies between different parts of your code more explicit.
In the following sections, we’ll explore how to work with global variables in Python functions, mindful of the potential issues and keeping in mind the best programming practices.
Using global variables in Python functions
Python makes it straightforward to access global variables within your functions. Let’s explore the syntax and grasp how to make the most of global variables in your Python programs.
The syntax for accessing global variables
The beauty of global variables lies in their accessibility — they can be accessed from any function within your program. To access a global variable inside a function, you use its name, just like you would with a local variable.
Here’s the basic syntax:
# Define a global variable
myGlobal = 10
def myFunction():
# Access the global variable
print(myGlobal)
myFunction() # Prints: 10
In this example, myGlobal
is a global variable. Inside myFunction
, we can print the value of myGlobal
without any additional keywords.
Examples of using global variables in functions
Now that we understand the basic syntax let’s look at a more practical example.
Suppose we are building a program that simulates a bank account. We could use a global variable to represent the account balance, which would need to be accessed and potentially modified by several functions:
# Define a global variable for the account balance
balance = 0
def display_balance():
# Access the global variable
print("Current balance: $", balance)
def deposit(amount):
# To modify the global variable, we need to declare it as global
global balance
balance += amount
print("Deposited: $", amount)
def withdraw(amount):
global balance
if amount > balance:
print("Insufficient funds")
return
balance -= amount
print("Withdrawn: $", amount)
# Test the functions
deposit(1000) # Deposited: $ 1000
withdraw(200) # Withdrawn: $ 200
display_balance() # Current balance: $ 800
In this code, balance
is a global variable representing the bank account balance. The deposit
and withdraw
functions declare balance
as global so they can modify it. The display_balance
function can directly access balance
to print it.
In the next section, we’ll dive deeper into how to modify global variables within functions. Remember, though convenient, global variables should be used judiciously to keep your code easy to understand, maintain, and debug.
Creating and modifying global variables within functions
One of Python’s strengths is its flexibility, which extends to controlling variables. Not only can you access global variables within a function, but you can also create and modify them. This section covers the techniques and considerations for declaring and altering global variables from inside a function.
How to declare global variables within a function
While it’s common to declare global variables at the top level of a script, Python also allows you to declare them within a function. You might want to do this when a variable should be accessible to multiple functions, but it is only relevant if a certain function is executed first.
You can declare a global variable inside a function using the global
keyword followed by the variable name. Once you declare a variable global, you can assign a value within the function. This value will then persist in the global scope after the function has been completed.
Here’s a basic example:
def set_global_var():
global myGlobal
myGlobal = 10
set_global_var()
# Now, we can access `myGlobal` from anywhere in our script
print(myGlobal) # Prints: 10
In this code, we declare myGlobal
as a global variable inside the set_global_var
function and assign it a value of 10. After we call set_global_var
, myGlobal
exists in the global scope and can be accessed normally.
Modifying global variables inside a function
Similarly, if you want to modify a global variable inside a function, you must first declare it as global within that function. After executing the function, you can change its value, which will persist globally.
# Define a global variable
myGlobal = 10
def modify_global_var():
global myGlobal
myGlobal += 5
modify_global_var()
# The global variable has been updated
print(myGlobal) # Prints: 15
In this code, we define myGlobal
as a global variable with a value of 10. Inside modify_global_var
, we declare myGlobal
as global and add 5 to its value. After calling modify_global_var
, the global myGlobal
has been updated to 15.
Note that without the global
keyword inside the function, Python would create a new local variable named myGlobal
, leaving the global myGlobal
unchanged.
Using the global keyword allows your functions to interact with the global scope when necessary while keeping their scope mostly isolated. This way, Python combines function scope's benefits with global variables' flexibility. However, as with all powerful tools, it's important to use them wisely to avoid making your code hard to understand or maintain.
Best practices and alternatives to global variables
While global variables have their uses, they can lead to hard-to-understand, test, and debug code, especially in larger applications. Thus, it’s critical to use them judiciously. Let’s discuss when to avoid using global variables and explore alternative strategies.
When to avoid using global variables
You should generally avoid using global variables when:
- The variable is only used by a single function or a small group of functions. In this case, passing the variable as an argument is better.
- The variable is being used to return a value from a function. A return statement is a much better tool for this job.
- The variable is being accessed or modified by multiple threads. This can lead to race conditions and other concurrency issues.
- The variable’s value is hard to predict because it is modified in many places. This makes your code hard to understand and debug.
Alternatives to global variables
There are several strategies for managing data within your programs that avoid the potential pitfalls of global variables. Let’s go over a few of them:
- Passing Variables as Arguments: The simplest alternative to global variables is to pass data to functions as arguments. This makes the data flow through your code much easier to follow and eliminates the risk of unintended side effects.
- Return Statements: Similarly, you can use return statements to get data from a function. Again, this makes the data flow more explicit and helps prevent bugs.
- Classes: Classes allow you to bundle data and the functions that operate on that data into a single unit called an object. An object's data (attributes) can be accessed and modified by its methods and isolated from the rest of your program.
- Closures: A closure is a function that has access to its scope, the scope of the outer function, and the global scope. It’s a way to carry some data (the variables from the outer function) every time you call the function.
Example of refactoring a function that uses global variables
Let’s refactor our earlier bank account example to use a class instead of a global variable:
class BankAccount:
def __init__(self):
self.balance = 0
def display_balance(self):
print("Current balance: $", self.balance)
def deposit(self, amount):
self.balance += amount
print("Deposited: $", amount)
def withdraw(self, amount):
if amount > self.balance:
print("Insufficient funds")
return
self.balance -= amount
print("Withdrawn: $", amount)
# Test the class
account = BankAccount()
account.deposit(1000) # Deposited: $ 1000
account.withdraw(200) # Withdrawn: $ 200
account.display_balance() # Current balance: $ 800
Instead of using a global balance
variable, we've defined a BankAccount
class. This class has an attribute balance
and methods to display the balance, deposit money, and withdraw money. When we create a BankAccount
object, its methods operate on its balance
attribute, isolated from the rest of our program.
In the final analysis, while global variables are a tool at your disposal in Python, they should be used sparingly and thoughtfully. Be sure to understand the potential issues and keep the principles of clean, maintainable code in mind when deciding how to structure your programs.
Global variables and multi-threading
Multithreading is a powerful feature in Python that allows your program to do multiple things simultaneously, improving performance and responsiveness. However, things can get tricky when it comes to using global variables in a multithreaded context. Let’s delve into this topic and explore potential issues and solutions.
A brief overview of multi-threading in Python
In Python, multithreading refers to the concurrent execution of more than one sequential set (thread) of instructions. In simpler terms, multithreading allows your program to do multiple things simultaneously.
For instance, one thread might be downloading a file from the internet, another thread could be processing a different file, and a third thread could be updating the user interface.
Python’s threading
module provides a simple interface for creating and managing threads. Despite the Global Interpreter Lock (GIL), which limits threads to running one at a time in standard Python, multithreading can still be beneficial for IO-bound tasks or for improving your programs' responsiveness.
Potential problems with global variables and threads
When working with multiple threads, global variables can quickly become a source of headaches. Here are some of the issues you might encounter:
- Race Conditions: If two threads access or modify a global variable simultaneously, it can lead to unexpected behavior. This is known as a race condition.
- Inconsistent State: Since threads can be scheduled to run at any time, a thread might be interrupted while updating a global variable, leaving the variable in an inconsistent state.
- Difficulty Debugging and Testing: The non-deterministic nature of thread scheduling makes bugs involving global variables and threads hard to reproduce and debug.
Strategies for dealing with these problems
Despite these challenges, there are strategies for safely using global variables in a multi-threaded environment:
- Locks: Python’s
threading
module provides aLock
class that allows you to synchronize threads. A lock can ensure that only one thread can access a global variable at a time, preventing race conditions. - Thread-Local Data: The
threading
module also provides alocal
class that allows you to create variables that are local to each thread, similar to how local variables work within functions. - Avoid Global Variables: In many cases, the best way to avoid the problems associated with global variables and threads is to avoid global variables altogether. Using queues or other thread-safe data structures, you can often redesign your program to pass data between threads.
Multithreading is a powerful tool but should be used judiciously, especially when global variables are involved. You can write safe, effective multithreaded Python code with the right knowledge and strategies.
Conclusion
Throughout this exploration, we have journeyed deep into the intricacies of using and creating global variables in Python. We’ve seen how these universal entities, accessible throughout our programs, can be both a useful tool and a source of potential challenges.
We started by understanding the scope of variables in Python, delving into how the ‘LEGB’ rule governs the accessibility and lifespan of our variables.
We then moved on to understanding the use cases for global variables and how they can play a crucial role in certain scenarios, such as sharing data between functions or maintaining state across function calls.
However, global variables have their complications. We explored potential problems, especially in multithreaded programs, where race conditions and inconsistent states can crop up.
We also looked at best practices and alternatives to using global variables, including passing variables as arguments, utilizing return statements, leveraging the power of classes, and implementing closures.
Remember, the strength of a great programmer lies in their ability to choose the right tool for the task at hand. Global variables, while powerful, should be used sparingly and wisely to ensure your code remains maintainable, reliable, and efficient.
In the quest for continual learning, you may wish to explore resources such as Python’s official documentation, online coding platforms, and community forums.
Keep experimenting and practicing with different scenarios to master the use of global variables in Python truly.
The key takeaway is that while global variables can be handy, they should be used carefully to understand their potential implications on your code’s readability, maintainability, and overall performance.
The judicious use of global variables and Python’s diverse set of features can lead to robust, efficient, and clean code, propelling you further on your journey as a Python developer.
Happy coding!