How To Automatically Protect Azure App Services
Creating a PowerShell script to manage access restrictions on app services
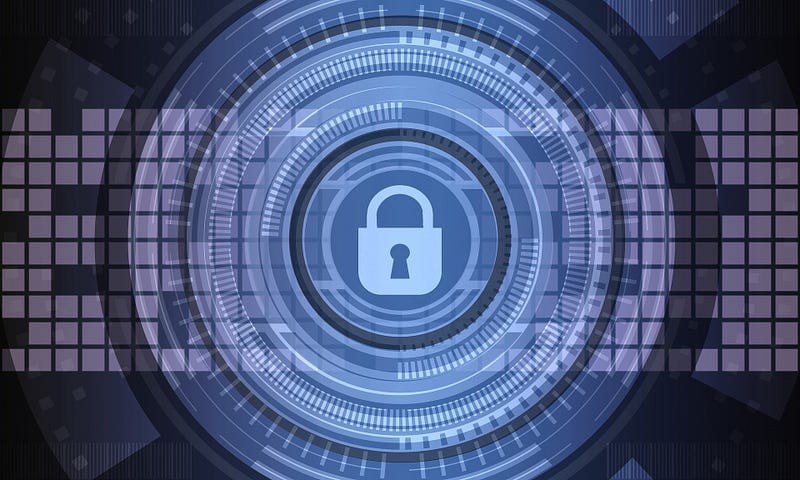
I think you’ll agree with me when I say that it is really tough to secure your applications.
Or is it?
It turns out it is, but we can make our lives easier by automating the more time-consuming and error-prone parts.
At our company, we use a lot of App Services in Microsoft Azure. An App Service is a Platform as a Service (PaaS) offering from Microsoft. We use it to host several services that are exposed via REST API’s.
These services and their APIs should not be publicly accessible. You can restrict App services access by specifying the allowed IP addresses. As we use many App Services, we wanted to manage these access restrictions automatically.
This article describes the solution we use to manage the access restrictions via a PowerShell script. You can find the scripts and the documentation in this GitHub repository.
Script Organization and Prerequisites
The solution consists of a single PowerShell module Manage-AccessRestrictions.psm1
and two PowerShell scripts, Remove-AccessRestrictions.ps1
and Add-AccessRestrictions.ps1
. The module contains the functions that are used by the two scripts. Both scripts can be called from the command line.
To use the scripts you need to install PowerShell 7.1.3, or higher. You also need to install the Azure Az PowerShell module which you can find here.
The scripts log information using Write-Information
. When the scripts are called you won’t see the logging. You have to add the -Verbose
option to make it visible.
Removing all existing access restrictions
Although we do not want to expose the App Services, sometimes it is necessary for a developer to access the service directly. For example, during troubleshooting.
What happens is that a developer adds an additional access restriction to access the App Service directly. They add their IP address manually via the Azure portal. When they are finished with troubleshooting, they remove their IP address.
However, sometimes, they forget this last step, removing their IP address.
Therefore, we have a PowerShell script that removes all the existing access restrictions and adds the default ones. This script runs once a day at night to reset all the access restrictions.
First, we will take a look at the function that removes all the existing access restrictions. The function Remove-AllAccessRestrictionsFromAppServices
as you can see below expects a single argument $ResourceGroupName
. This argument identifies the resource group that contains the App Services. A resource group in Azure is a container for resources.
The function first retrieves all the app services in the resource group via Get-AzWebApp
. This is a function from the Azure Az PowerShell module. Then we iterate over all the app services and call the Remove-AccessRestrictionsFromAppService
function.
The Remove-AccessRestrictionsFromAppService
a function that you see below removes all the access restrictions from a single App Service. The first thing the function does is retrieve the existing access restriction via Get-AzWebAppAccessRestrictionConfig
. The result contains all the rules that exist on the App Service. The function then removes the rules using Remove-AzWebAppAccessRestrictionRule
.
As an App Service can contain deployment slots, we also need to remove the access restrictions on the deployment slots. This happens on lines 12–22.
These functions are used by a single script called Remove-AccessRestrictions.ps1
which you can start from the command line.
Calling the Remove Access Restrictions script
You can use the Remove-AccessRestrictionsFromAppService
by calling the Remove-AccessRestrictions.ps1
script via the PowerShell command-line.
The script has a single required parameter called ResourceGroupName
. It first imports the Manage-AccessRestrictions
module and then calls LoginToAzure
. This shows the Microsoft login screen to enter your credentials. After logging in, the function Remove-AccessRestrictionsFromAppServices
is called.
See the implementation of the Remove-AccessRestrictions.ps1
script below.
Adding Access Restrictions
The script to add the access restrictions works using an input file that specifies in JSON what IP addresses to add to the access restrictions. See below for an example of the file.
The rule name is an identification of the rule. You should set this to something that you recognize. The IP address is the address that can access the App Service. The latest field, priority, indicates the order in which Azure evaluates the rules.
Only the IP addresses in this file will have access to the App Services in the specific Resource group when the script finishes.
The Add Access Restrictions script
The add access restriction script consists of two parts, the first part retrieves all the App Services from the given resource group, reads the JSON file, and validates the exclude list. See below the first part of the script.
On line 7, all the app services are retrieved from the given resource group using the Get-AzWebApp
function. Then on line 9, a special function is called that validates if the app services to exclude are actually present in the resource group. This prevents running the script when you made a typo in one of the app services that you want to exclude.
On the last line, 18, the JSON file is read and stored in the variable $ipRules
.
The second part of the script iterates over the App Services from the resource group and sets the access restrictions. See below for this second part of the script.
The second part starts with the label :nextAppService
. This label is used when an App Service is found in the exclude list. On line 10, we jump directly to the next App Service.
We check if the $RemoveExistingRules
flag is true. If so, we remove the existing rules by calling Remove-AccessRestrictionsFromAppService
. Which is the same function that is used by the Remove Access Restrictions script.
Finally, the script iterate over all the records from the JSON file and for each record calls the Add-AccessRestrictionToAppService
function.
The Add-AccessRestrictionToAppService
function uses the Add-AzWebAppAccessRestrictionRule
to add a new restriction rule to the App Service, see below. It also calls Update-AzWebAppRestrictionConfig
with the argument -ScmSiteUseMainSiteRestrictionConfig
. This means the SCM (Source Control Manager) site of your App Service will use the same access restrictions.
The function will also set the same access restrictions on all the staging slots it finds with the App Service.
You can execute the Add-AccessRestrictionsToAppServices
by using the Add-AccessRestrictions.ps1
from the command line.
Calling the Add Access Restrictions script
You can use the Add-AccessRestrictionsToAppService
function by calling the Add-AccessRestrictions.ps1
script via the PowerShell command line.
The script has two required and two optional parameters. The required parameters are called JsonFilename
and ResourceGroupName
. The two optional parameters can be used to remove the existing access restrictions and to exclude some App Services.
It first imports the Manage-AccessRestrictions
module and then calls LoginToAzure
. This shows the Microsoft login to enter your credentials. Then the function Add-AccessRestrictionsToAppServices
is called.
See the implementation of the Add-AccessRestrictions.ps1
script below.
Conclusion
Security is tough! But you can make your life easier by automating the more time-consuming and error-prone parts. We use the PowerShell scripts from this article to reset the access restrictions on our App Services every night.
A thing we added was to also add Azure DevOps pipelines to the access restrictions. Otherwise, we could not deploy from the Azure DevOps pipeline to our App Services. We used the service tag of the access restrictions with the Azure DevOps pipeline.
Suppose you need more or different rules for your infrastructure. Make sure to look at the functions and documentation of the Azure Az PowerShell module. I am almost certain that there are functions in this module that can help.
Now, this is certainly not the only way to secure your Azure App Services. There are other options, such as placing your App Services in a virtual network. This does affect the type of App Services you can use and can increase costs.
Until now, whitelisting resources to access the App Service works for us. You can find the scripts and their documentation in this GitHub repository.
Thanks for reading, and remember never to stop learning.