Demystifying Python’s Magic Methods — A Deep Dive
Unlocking the power of dunder methods for more expressive and efficient code
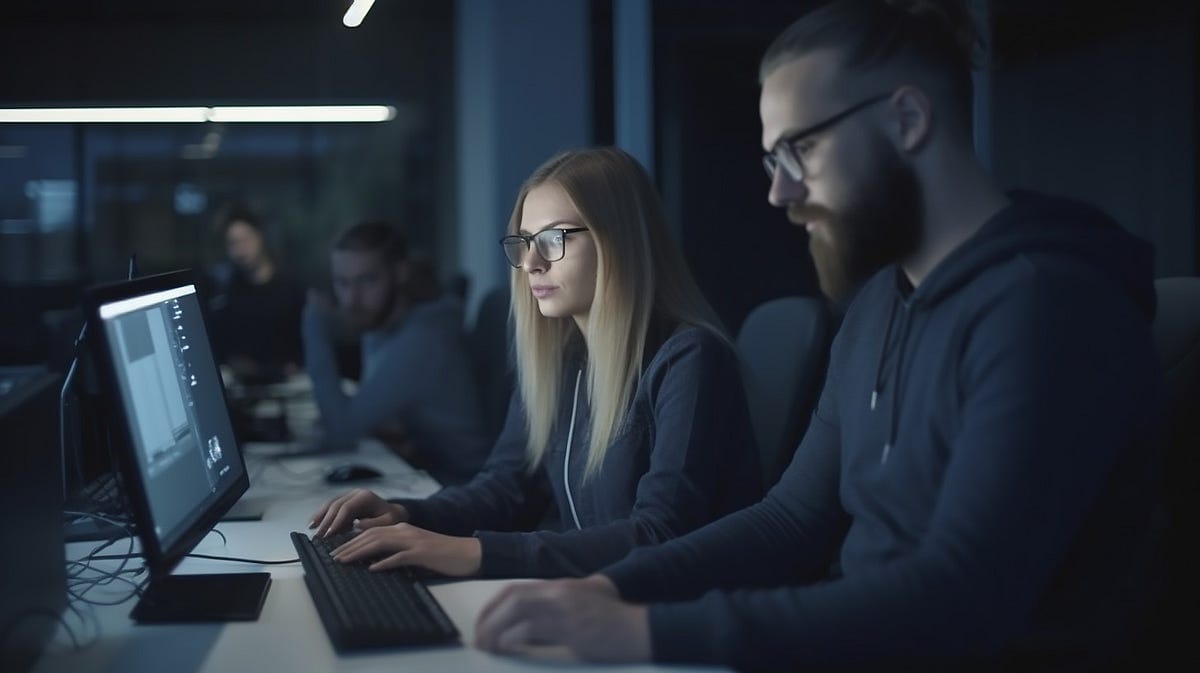
At QuantumPay Solution, two developers, Amara and Kaden, were assigned to build a complex system for a renowned banking institution. The system’s core required a mechanism to handle complex financial transactions, specifically, the arithmetic of monetary amounts.
Their codebase had a Money
class representing a monetary value, with attributes for currency and amount. One of the frequent operations they had to perform was adding two Money
objects together. Their initial approach was a bit cumbersome - they had a custom method to add the amounts and ensure the currencies were the same:
def add_money_objects(money1, money2):
if money1.currency != money2.currency:
raise ValueError("Currencies do not match!")
return Money(money1.currency, money1.amount + money2.amount)
Kaden, an experienced Python developer, saw an opportunity to introduce Amara, a new developer on the team, to a concept that could simplify their code — Python’s magic methods.
“Hey, Amara,” Kaden suggested, “what if I told you we could add two Money
objects just like we add two integers or floats in Python? We can make our Money
class behave like a built-in data type."
Amara, intrigued, asked how. Kaden then introduced her to the __add__
magic method.
Kaden explained, “If we define an __add__
method in our Money
class, we can use the '+' operator directly on our objects. This magic method is automatically called when we use '+'. It makes our code cleaner and easier to understand."
Following Kaden’s explanation, they refactored their Money
class:
class Money:
def __init__(self, currency, amount):
self.currency = currency
self.amount = amount
def __add__(self, other):
if self.currency != other.currency:
raise ValueError("Currencies do not match!")
return Money(self.currency, self.amount + other.amount)
With this change, they could add two Money
objects as quickly as money1 + money2
, just like built-in Python data types.
This was a revelatory moment for Amara. She marveled at how Python’s magic methods made their code more elegant and intuitive, and was eager to discover what other magical methods could do.
In this article, we’re set to demystify Python’s magic methods. Together, we’ll explore their powers and applications and how they can be wielded to make your code more ‘Pythonic.’
By the end, you’ll have a comprehensive understanding of these hidden gems and know how to apply them to transform your programming practice.
The Basics
Magic Methods, often called ‘dunder’ methods from the double underscores (__), are a set of special methods in Python classes that provide a host of essential functionalities.
While they’re as diverse as the programming language itself, here are a few common ones you may already be familiar with:
__init__
: Acts as the constructor for your class, initializing new objects.__str__
: Returns a human-readable string representation of your object.__repr__
: Unlike str, repr provides a more detailed and developer-friendly string representation of your object. Mainly used for debugging purposes.
What sets these methods apart? Besides the fact that they’re all bookended with double underscores (__), these magic methods are ‘special’ because Python calls them automatically under specific circumstances.
For instance, when we use the str()
function on an object, Python internally calls the object's __str__()
method. We never explicitly call obj.__str__()
; Python does this behind the scenes.
Operator overloading
Python allows you to redefine the behavior of built-in operators using operator overloading. This means you can customize how operators, like addition, subtraction, multiplication, division, etc., work on objects of your custom classes. This powerful feature is achieved by implementing special methods, often referred to as “magic methods” or “dunder methods” (short for “double underscore methods”).
Magic methods are unique, with predefined names that Python recognizes and automatically calls in response to a certain operator or built-in function invocations. You can define custom behavior for operators in your classes by implementing specific magic methods.
Here are some commonly used magic methods for operator overloading:
__add__(self, other)
: Implements the addition operator+
.__sub__(self, other)
: Implements the subtraction operator-
.__mul__(self, other)
: Implements the multiplication operator*
.__truediv__(self, other)
: Implements the true division operator/
.__floordiv__(self, other)
: Implements the floor division operator//
.__mod__(self, other)
: Implements the modulo operator%
.__pow__(self, other[, modulo])
: Implements the exponentiation operator**
.__eq__(self, other)
: Implements the equality operator==
.__lt__(self, other)
: Implements the less than operator<
.__gt__(self, other)
: Implements the greater than operator>
.__str__(self)
: Returns a string representation of the object.__repr__(self)
: Returns a string representation that can be used to recreate the object.
Here’s an example to demonstrate the usage of magic methods for operator overloading:
class Vector:
def __init__(self, x, y):
self.x = x
self.y = y
def __add__(self, other):
return Vector(self.x + other.x, self.y + other.y)
def __sub__(self, other):
return Vector(self.x - other.x, self.y - other.y)
def __mul__(self, scalar):
return Vector(self.x * scalar, self.y * scalar)
def __str__(self):
return f"Vector({self.x}, {self.y})"
# Create two Vector objects
v1 = Vector(2, 3)
v2 = Vector(4, 5)
# Addition
result = v1 + v2
print(result) # Output: Vector(6, 8)
# Subtraction
result = v1 - v2
print(result) # Output: Vector(-2, -2)
# Scalar multiplication
result = v1 * 2
print(result) # Output: Vector(4, 6)
In the above example, the Vector
class defines magic methods __add__
, __sub__
, and __mul__
to overload the addition, subtraction, and multiplication operators, respectively. These methods allow the objects of the Vector
class to be added, subtracted, and multiplied with other Vector
objects or scalars.
By implementing magic methods appropriately, you can provide intuitive and meaningful behavior for operators on your custom classes, making your code more expressive and readable.
Remember that operator overloading should be used judiciously and in a way that makes logical sense for your class and its objects.
Comparison Magic Methods
Magic methods in Python allow for specific built-in behavior to be customized, such as comparison between objects. Here are the six magic methods that you can use for comparison:
__eq__
: This is the equality magic method. It allows us to use the==
operator to compare two objects.__ne__
: This is the not-equal-to magic method. It allows us to use the!=
operator to compare two objects.__lt__
: This is the less-than magic method. It allows us to use the<
operator to compare two objects.__gt__
: This is the greater-than magic method. It allows us to use the>
operator to compare two objects.__le__
: This is the less-than-or-equal-to magic method. It allows us to use the<=
operator to compare two objects.__ge__
: This is the greater-than-or-equal-to magic method. It allows us to use the>=
operator to compare two objects.
Here is an example of how to use these magic methods in a Python class:
class Person:
def __init__(self, age):
self.age = age
def __eq__(self, other):
if isinstance(other, Person):
return self.age == other.age
return False
def __ne__(self, other):
return not self.__eq__(other)
def __lt__(self, other):
if isinstance(other, Person):
return self.age < other.age
return False
def __gt__(self, other):
if isinstance(other, Person):
return self.age > other.age
return False
def __le__(self, other):
if isinstance(other, Person):
return self.age <= other.age
return False
def __ge__(self, other):
if isinstance(other, Person):
return self.age >= other.age
return False
With these magic methods implemented, you can now compare Person
instances just like you would with integers or other comparable types:
p1 = Person(20)
p2 = Person(25)
print(p1 == p2) # False
print(p1 != p2) # True
print(p1 < p2) # True
print(p1 > p2) # False
print(p1 <= p2) # True
print(p1 >= p2) # False
Note that the other
argument in each method refers to the object that self
is being compared to. Also, we check if other
is an instance of Person
before proceeding with the comparison to prevent errors when comparing a Person
to an incompatible type.
Advanced Magic Methods
This sections covers more complex magic methods, such as those that enable objects to behave like sequences or mappings, or those that enable context management (__enter__
and __exit__
).
Sequence Magic Methods
Python provides magic methods to make objects behave like sequences (__len__
, __getitem__
, __setitem__
, __delitem__
, __contains__
):
__len__(self)
: This method should return the length of the object, which can be any integer >= 0.__getitem__(self, key)
: This method should return the value of the item at the position specified bykey
.__setitem__(self, key, value)
: This method should set the value of the item at the position specified bykey
tovalue
.__delitem__(self, key)
: This method should remove the item at the position specified bykey
.__contains__(self, item)
: This method should return True ifitem
is in the object, and False otherwise.
Here’s an example of a simple Container
class that uses these methods:
class Container:
def __init__(self):
self.data = []
def __len__(self):
return len(self.data)
def __getitem__(self, index):
return self.data[index]
def __setitem__(self, index, value):
self.data[index] = value
def __delitem__(self, index):
del self.data[index]
def __contains__(self, item):
return item in self.data
Context Manager Magic Methods
Python also provides magic methods to enable objects to function as context managers (__enter__
, __exit__
):
__enter__(self)
: This method is run when execution flow enters the body of thewith
statement. It returns the resource to manage.__exit__(self, exc_type, exc_val, exc_tb)
: This method is run when execution flow leaves the body of thewith
statement. It can be used to handle exceptions, clean up resources, or do other closing actions.
Here’s an example of a ManagedFile
class that uses these methods to manage a file resource:
class ManagedFile:
def __init__(self, filename):
self.filename = filename
def __enter__(self):
self.file = open(self.filename, 'r')
return self.file
def __exit__(self, exc_type, exc_val, exc_tb):
if self.file:
self.file.close()
This class can be used like this:
with ManagedFile('hello.txt') as f:
print(f.read())
The ManagedFile object is being used as a context manager in this code. The __enter__
method is called at the start of the with
statement, and the __exit__
method is called at the end.
Best Practices
While magic methods can be a potent tool in Python, it’s important to use them responsibly and carefully. Here are some best practices to follow:
1. Stick to the intended purpose of magic methods
Each magic method is designed to perform a specific task or behave a certain way. It’s best to adhere to the intended behavior of these methods to avoid confusion and keep your code transparent and predictable. For instance, the __eq__
method should always be used to check for equality, not to perform some unrelated side-effect.
2. Avoid magic method overuse
Magic methods should be used judiciously. The unnecessary use of magic methods can make your code more complicated, less readable, and harder to debug. If you use many magic methods, consider whether there might be a more straightforward way to achieve your goal.
3. Ensure magic methods are correctly implemented
If you choose to implement a magic method, ensure it is correctly implemented. This includes correctly handling the arguments given to the method and returning the expected values. If you implement one magic method for a concept, such as a comparison, consider implementing all appropriate magic methods to ensure consistency and predictability.
4. Use magic methods to make your code more Pythonic
One of the main benefits of magic methods is that they allow your custom objects to behave like built-in Python objects. This can make your code more intuitive and Pythonic. For example, implementing the __len__
method allows you to use the built-in len()
function on your object.
5. Implement __repr__
for debugging
The __repr__
method returns a string that describes an instance of a class. It is beneficial for debugging and should be implemented in every class. It should strive to be unambiguous and match the syntax needed to recreate the object.
6. Implement context management methods when applicable
If your class manages a resource that needs to be set up and then torn down (like files, network connections, etc.), consider implementing __enter__
and __exit__
methods so that your class can be used with a with
statement. It's an easy way to ensure that resources are correctly cleaned up, even if an error occurs.
By following these best practices, you can ensure that your use of magic methods is effective, responsible and contributes to the quality and readability of your code.
Conclusion
Python’s magic methods provide a powerful way to customize your objects, allowing them to emulate built-in types, interact with operators, handle context management, and much more.
This helps in designing more robust and expressive classes and contributes towards writing more ‘Pythonic’ code, blending seamlessly with idioms and patterns of the language.
However, with this power comes responsibility. It’s important to remember that these methods should be used judiciously and for their intended purpose to maintain readability and avoid unnecessary complexity in your code.
This article introduced the basics and some advanced uses of magic methods. But Python has even more magic methods for you to explore, including those for unary operators, compound assignment operators, and others. Each has its unique functionality and adds another tool to your Python toolbelt.
I encourage you to delve deeper into Python magic methods. Whether building a simple script or a complex system, these techniques can help you write cleaner, more efficient, and more intuitive code. Python’s magic methods truly open up a whole new level of programming.
Happy coding!
More content at PlainEnglish.io.
Sign up for our free weekly newsletter. Follow us on Twitter, LinkedIn, YouTube, and Discord.